Table of contents
In std, you can use cerr(1)Printing exception
std::cin >> a;
if(a == 0){
std::cerr << "division by zero!" << std::endl;
}
Program Termination
1. return
2. exit()
Using exit()(2)Function exits the program, exit(0)(3)It means that the program runs successfully and exits normally.
int main() {
int a;
cin >> a;
if (a == 0) {
cerr << "division by zero!" << endl;
exit(-1);
}
cout << 10 / a << endl;
return 0;
}
and return(4)The difference is: return is similar to keywords such as continue and break, which are used to return function values; while exit is to exit the program directly. In the main function, there is basically no difference between the two, and both can be used to end the program.
3. abort()
abort()(5)Function can also be used to exit the program.
Difference between abort() and exit()
#include <iostream>
using namespace std;
class Function{
public:
Function(){
cout << "Function constructor called" << endl;
}
~Function(){
cout << "Function destructor called" << endl;
}
};
int main(){
Function f1;
exit(0);
return 0;
}
Output:
Function constructor(6) called
#include <iostream>
using namespace std;
class Function{
public:
Function(){
cout << "Function constructor called" << endl;
}
~Function(){
cout << "Function destructor called" << endl;
}
};
Function f1;
int main(){
exit(0);
return 0;
}
Output:
Function constructor called
Function destructor(7) called
It can be seen that the exit() function can call the destructor, but the automatic object(8)The destructor of the object will not be called. (Automatic object: an object allocated in a subroutine or block, seehttps://www.ibm.com/docs/en/xl-fortran-linux/16.1.0?topic=objects-automatic)
The abort() function does not call the destructor at all.
Assertion
Requires #include(9)
usage:
#include<iostream>
#include<cassert>
using namespace std;
int main() {
assert(1 == 0);
return 0;
}
PS D:\2023-2024\course-oop\test> .\test.exe
Assertion failed: 1 == 0, file check.cpp, line 5
If the assertion fails, the program stops immediately.
Assertions can be ignored using #define NDEBUG.
Exceptions and Errors
Exception handling in C++ includes: try(10), throw(11), catch(12)
You can use try to define a block of code that you can test for errors when it is executed.
usage:
#include <iostream>
int main(){
std::string str("foo");
try{
str.at(10); // 抛出 out_of_range 异常
}catch(const std::out_of_range& e){
std::cout << "out_of_range: " << e.what() << std::endl; // what() 返回异常的描述信息
// 这里捕获的是 std::exception 的子类 std::out_of_range 异常
}catch(const std::exception& e){ // 当然,也可以捕获其他异常
std::cout << "exception: " << e.what() << std::endl;
}
return 0;
}
Real-world use cases:
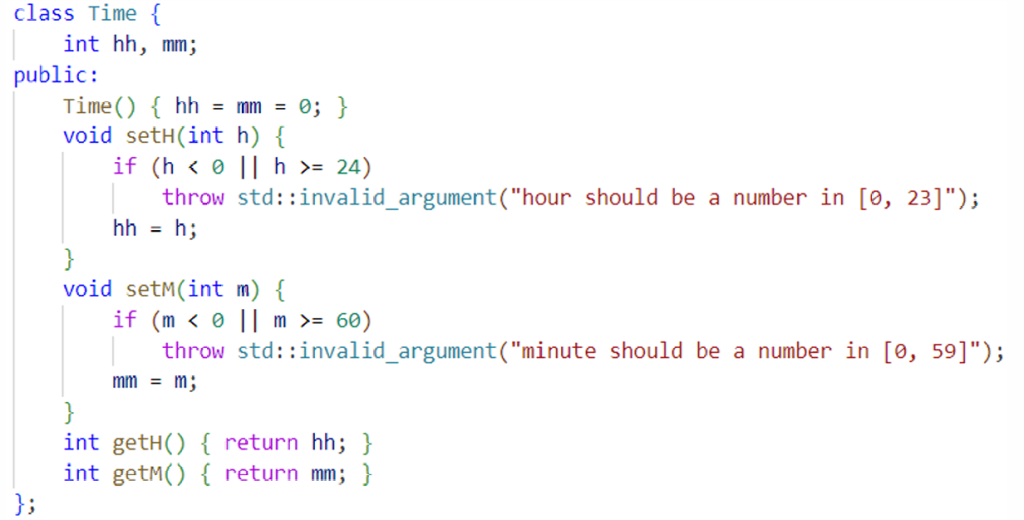
Here, we define a time class and need to ensure that the hours and minutes are in the correct format. So when the time input is not compliant, an exception can be thrown and caught elsewhere.
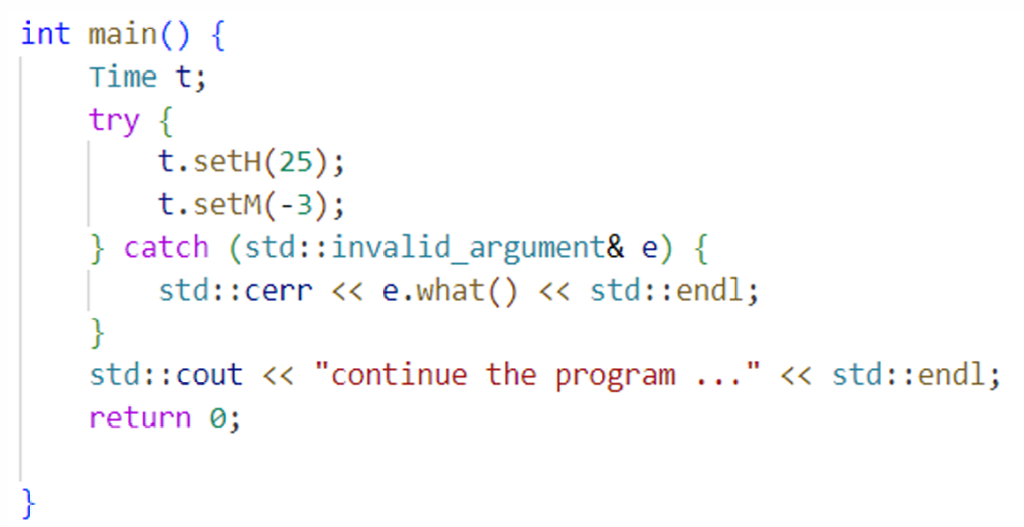
Catch has one parameter. When the parameter in catch is the same as the parameter thrown by throw, the content in the catch code block will be executed.
You can use multiple catch to catch different exceptions
int main(){
try{
}catch(type1& e1){
}catch(type2& e2){
}catch(...){
//如果不清楚错误类型,可以使用catch(...)代替
}
return 0;
}
For details, please see:https://www.w3schools.com/cpp/cpp_exceptions.asp
Comments NOTHING